Clean code is vital for strong applications, preventing harmful bugs that can hurt performance and drive users away. While QA testing is common, both testers and developers tackle debugging. Simple strategies work for different software. Bugs are normal and need various tools to fix. Debugging, important but tough, relies on quick error spotting and efficient fixing, saving time and resources. This article explores key debugging ideas, tools, and methods, showing how they fit into software development.
Debugging is the process of finding flaws, defects, or problems in the software or the lines of code that cause the application to behave erratically or crash, and removing them. In the lifecycle of software development, debugging is a crucial step. While it’s a part of QA testing, QA is a more thorough step that includes verifying other areas of quality, such as performance and usability, rather than only discovering bugs.
A product can never be completely error-free. The following strategies can be used by a developer to fix a bug in a program after first identifying it.
1. Ask yourself the right question
By describing the issue and asking yourself questions about it, you may begin the debugging process. For example:
- What exactly does the program need to do?
- What does it do in reality?
- What problems have you discovered?
- Have you ever faced issues similar to these before?
- How did you make them better?
- Where do you believe the bugs appeared, and why?
Asking these questions frequently will encourage you to develop a hypothesis about the nature of the errors, enabling you to identify their primary source and fix it.
2. Read Documentations and Error Messages properly
When reading error messages, developers frequently make a mistake. They become anxious and frequently scan the messages only partially. The majority of the time, messages contain the answers to errors. Sometimes, errors occur due to not fully understanding the libraries or frameworks you’re using. Reading the documentation can provide insights into proper usage.
These messages give you specific information about the status of the product. If you’re having trouble understanding the error messages, talk to your seniors because they may have experienced the same thing, or try searching online.

When examining the documentation for the React JS framework concerning the provided image, it becomes evident that custom hooks cannot be invoked within a regular function.
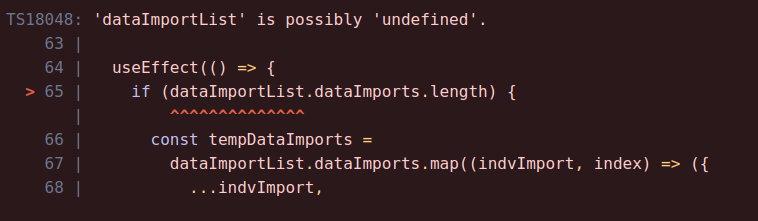
From the image, we can see that the error occurs because “dataImportList” is undefined. To fix this, we can make it simpler by defining the variable.
const dataImportList = {
dataImports: []
}
// OR
useEffect(( ) => {
if(dataImportList?.dataImports?.length) {
// … remaining code
}
}. [])
3. Use a Debugger
A debugger, referred to as a debugging tool or debugging mode, proves highly effective for detecting and rectifying bugs. To utilize this tool, you insert it into the program and initiate it, enabling real-time error identification. It’s possible to halt the program during execution to precisely examine and address any ongoing problems.
import React, { useState } from “react”;
const Add = () => {
const [number, setNumber] = useState({ first_number: 0, second_number: 0 });
const [sum, setSum] = useState(0);
const { first_number, second_number } = number;
const onChange = (event) => {
const { name, value } = event.target;
setNumber({ …number, [name]: value });
};
const onClick = () => {
debugger // pauses the code execution
const tempSum= Number(first_number) + Number(second_number)
setSum(tempSum);
}
return (
<div>
Sum of
<input type=”number” name=”first_number” value={first_number} onChange={onChange} />
<input type=”number” name=”second_number” value={second_number} onChange {onChange} />
is {!!sum && <h2>{sum}</h2> }
<br />
<button onClick={onClick}>Add</button>
</div>
);
};
export { Add };
Additionally, you can sequentially execute each line of code to comprehend the program’s flow. For browser-based debugging, access the inspect section to locate the play button for complete code execution, or the next step button to execute each line individually.
4. Log everything
Developers mostly employ this approach to display values in their console. This aids in recognizing whether a function or a class component is obtaining the correct inputs and producing the anticipated outcomes. These logged statements facilitate the discovery of the underlying issue, particularly in cases where a variable holds unexpected values.
Taking the example of above code snippet, we can use log as follows:
const onClick = () => {
console.log(“first number”, first_number, typeof first_number);
// first number, 4, string
console.log(“second number”, second_number, typeof first_number);
// second number, 8, string
// since the fist_number and second_number are string and trying to add them
// with plus (+) sign only concatenates them.
// So we convert them to numbers before adding them
const tempSum = Number(first_number) + Number(second_number);
console.log(“sum”, tempSum, typeof tempSum);
// sum, 12, number
setSum(tempSum);
};
4. Binary Search
When you’re dealing with a big and frustrating code problem, you can try taking out one line of code at a time. Keep doing this until you discover the specific thing that’s causing the issue or making the program crash.
In conclusion, clean code is imperative for robust software, as it prevents performance-harming bugs and ensures a positive user experience. Debugging, a vital step in software development, involves identifying and resolving flaws. Key strategies for effective debugging include asking the right questions, reading documentation and error messages thoroughly, utilizing debuggers, logging values, and employing binary search techniques. These methods collectively contribute to efficient debugging, saving time and resources in software development. Remember, perfect software may not exist, but diligent debugging can get us closer to it.