Verdaccio is a lightweight, private npm registry designed to help teams and organizations manage and share npm packages with ease. By hosting your own packages, you maintain full control over your registry and package distribution. Here’s a concise guide to get you started with Verdaccio and creating a TypeScript-based npm package.
docker run -it --rm --name verdaccio -p 4873:4873 verdaccio/verdaccio
Running Verdaccio using Docker
Running using docker compose:
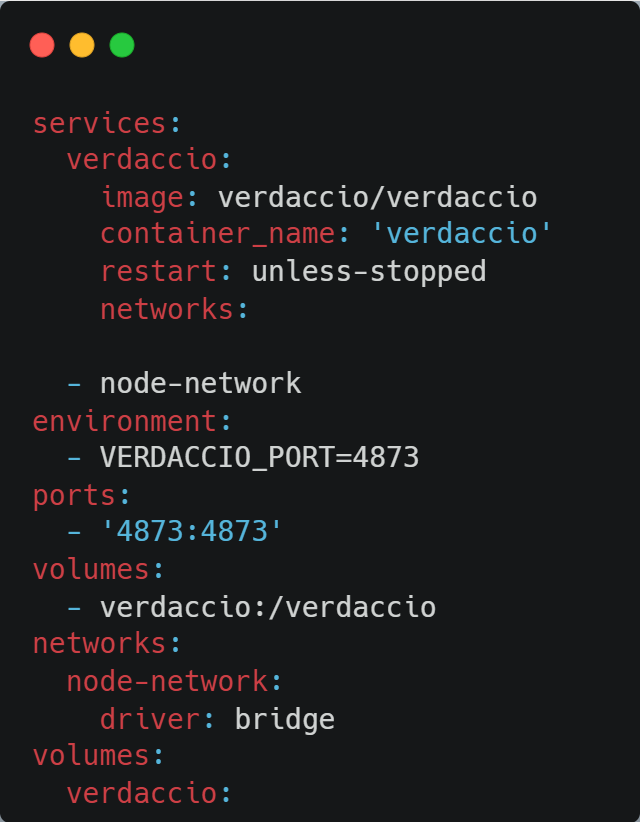
Run docker compose:
docker-compose up -d
When the container gets started, config.yaml is created in volume which can be used to modify configurations. Also data and htpasswd is created inside verdaccio container in path /verdaccio/storage . data consists of saved packages while htpasswd consists of users information.
Getting Started with Verdaccio
1. Install Verdaccio
Install Verdaccio globally with npm:
npm install -g verdaccio
2. Run Verdaccio
Start the Verdaccio server locally:
verdaccio
The server will be accessible at http://localhost:4873. Alternatively, you can deploy Verdaccio on a dedicated server for remote access.
3. Configure npm
Point npm to your Verdaccio registry:
npm set registry https://registry.npmjs.org/
To revert to the default npm registry:
npm set registry http://localhost:4873/
Creating and Publishing Your Package
Step 1: Initialize Your Package
Navigate to your project directory and initialize npm:
npm init
Step 2: Set Up TypeScript
Install TypeScript and type definitions:
npm install typescript @types/node --save-dev
Initialize a TypeScript configuration:
npx tsc --init
Step 3: Write Your Code
Create an index.ts
file in your project directory. This will serve as the main entry point for your package.
Step 4: Configure the Build Process
To publish our component on npm, there are some key steps to follow. Currently, we only have the source code, which we don’t want to upload as it isn’t optimized or minified. Instead, we’ll use the tsup tool to generate the build files. Tsup is a straightforward and efficient tool powered by esbuild, a highly performant bundler known for being significantly faster than alternatives like Rollup or Webpack.
1. Install tsup:
npm install -D tsup
2. Create a tsup.config.json file:
{ "splitting": true, "sourcemap": true, "clean": true, "dts":true, "outDir": "dist", "minify": true, "entry": [ "src/index.ts" ], "format":["esm", "cjs"] }
Update package.json with a build script:
{ “scripts”: { “build”: “tsup” } }
Step 5: Customize package.json
Prepare your package for deployment by specifying output paths:
{ "main": "dist/index.js", "types": "dist/index.d.ts", "module": "dist/index.mjs", "files": [ "dist" ] }
Publishing Your Package
1. Build Your Code: specifying output paths:
npm run build
2. Publish to Verdaccio: If you’re publishing to your Verdaccio registry:
Add a user:
npm adduser --registry http://localhost:4873
Login:
npm login --registry http://localhost:4873
Publish:
npm publish --registry http://localhost:4873
Publish to npm (optional): Log in and publish:
npm login npm publish
Comparing Verdaccio to Other Private npm Registries
When it comes to hosting private npm registries, several options are available. Here’s how Verdaccio stacks up against other popular solutions:
1. Verdaccio
Key Features:
- Lightweight and Easy Setup: Minimal configuration and fast setup process. Ideal for small teams or individual developers
- Open Source: Completely free and community-driven
- Local and Cloud Deployment: Can run locally for development or on servers for production
- Caching: Acts as a caching proxy for npm packages, reducing external requests
- Authentication and Permissions: Offers flexible user authentication methods (e.g., htpasswd, LDAP)
- Customizability: Extensible with plugins for logging, authentication, and more
Use cases:
- Best suited for developers who need a simple, fast, and cost-effective private registry solution
2. npm Enterprise
Key Features:
- Official Solution: Fully managed by npm, Inc., with tight integration into the npm ecosystem
- Team Management: Advanced access controls, organization-level package management
- Security: Enterprise-grade security with automated vulnerability scanning
- Private Scope: Allows packages to be scoped specifically for your organization
Limitations :
- Expensive subscription plans, making it less ideal for small teams or independent developers.
- Fully managed, with less control over the registry infrastructure
Use cases:
- Suited for large organizations with a budget for enterprise solutions
3. JFrog Artifactory
Key Features:
- Multi-Language Support: Hosts npm packages alongside other artifacts like Docker images, Maven, and Python packages
- Enterprise-Grade Features: High availability, scalability, and comprehensive access controls.
- Advanced Caching: Universal artifact caching and proxying
- Integration: Supports CI/CD tools like Jenkins, GitHub Actions, and Azure DevOps
Limitations :
- Complex setup and higher resource requirements compared to Verdaccio
- Premium features locked behind paid plans
Use cases:
- Ideal for organizations managing multiple package types and needing robust CI/CD integration
4. Azure Artifacts
Key Features:
- Cloud-Native: Fully integrated with the Azure DevOps ecosystem
- Universal Packages: Supports npm, Maven, NuGet, Python, and more
- Pay-As-You-Go: Pricing based on storage and bandwidth usage
- Team Collaboration: Seamless integration with Azure Pipelines and Boards
Limitations :
- Dependent on Azure DevOps ecosystem
- Not as customizable or lightweight as Verdaccio
Use cases:
- Best for teams already using Azure DevOps for development and deployment
5. GitHub Packages
Key Features:
- Built-In GitHub Integration: Directly tied to GitHub repositories for easy access
- Free for Public Repositories: No additional cost for hosting public packages
- Access Control: Granular permissions based on repository settings
Limitations :
- Limited to GitHub-hosted projects
- No standalone registry, as it’s tightly coupled with GitHub
Use cases:
- Ideal for developers already leveraging GitHub for code hosting and collaboration
Verdaccio in Perspective
Verdaccio stands out as a lightweight, free, and easy-to-use solution for hosting private npm registries. While it may lack some of the advanced enterprise features of solutions like Artifactory or npm Enterprise, its simplicity and flexibility make it an excellent choice for small-to-medium teams or solo developers.
If you’re looking for a straightforward way to manage npm packages without unnecessary complexity or costs, Verdaccio is a top contender.
Conclusion
With Verdaccio, managing private npm packages is seamless, and using TypeScript ensures robust, type-safe code. By following this guide, you can set up your own registry, create optimized packages, and share them efficiently.