Building upon the solid foundation of the last article, “Scoping to Data Preprocessing,” where we thoroughly explored the importance of scoping, as well as cleaning, transforming, and preparing data, we now begin the crucial phase of training and evaluating , and error analysis our machine learning models. This is where the rubber meets the road as our models are tested to learn from the data and make accurate predictions.
Armed with a well-prepared dataset, we will now delve into the intricacies of training algorithms, exploring various techniques to optimize model performance. We will also discuss the importance of evaluation metrics and how to select the right ones to assess a model’s effectiveness, along with techniques for analyzing errors.
Model Training : The Heart of Machine Learning
Model training is the process of teaching a machine learning algorithm to recognize patterns and make predictions based on the provided data. It involves selecting an appropriate model architecture, feeding it with the prepared dataset, and iteratively refining the model’s parameters through optimization techniques.
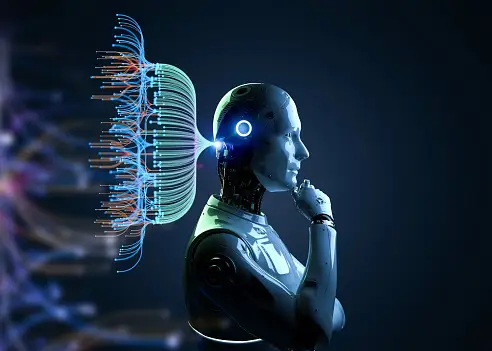
Key Steps in Model Training:
1. Model Selection:
- Choose a Model Architecture: Select a suitable model type based on the nature of your task (e.g., classification, regression, clustering). Consider factors like complexity, interpretability, and computational resources.
- Hyperparameter Tuning: Experiment with different hyperparameters (e.g., learning rate, regularization strength) to optimize the model’s performance.Check here hyperparameter-tuning for more detailed information.
2. Training the Model:
-
Feed Data: Input the training dataset into the model (e.g., an image, a text, or a numerical vector).
Example: For an image classification task, we might have a dataset of images of animals (cats, dogs, etc.). Each image would be converted into a numerical format (such as pixel values) that the model can understand. For a text classification task, the input might be sentences that are converted into word embeddings or numerical vectors using techniques like Tf–idf or Word2vec .
llustration:
- Image of a cat:
[0.2, 0.3, 0.5, ...]
(flattened pixel values) - Text of a sentence:
["the cat sat on the mat"]
→[0.1, 0.4, ...]
(numerical representation)
- Image of a cat:
-
Forward Pass: The model processes the input data to produce predictions.The input data is passed through a series of layers, each applying a mathematical transformation.
Example: In a neural network for image classification, the first layer might apply convolutional filters to detect edges in the image. The output of this layer is then passed to the next layer, which may apply pooling to reduce dimensionality. Eventually, the output layer produces a probability distribution over the classes (e.g., cat or dog).
Illustration:
- Input image vector:
X
- Layer 1 (Convolution):
Y1 = Conv(X, W1) + b1
- Layer 2 (Activation):
Y2 = ReLU(Y1)
- Output layer:
Predictions = Softmax(Y2)
- Input image vector:
- Backward Pass (Back-propagation): After obtaining predictions, we compare them to the actual labels to calculate the error (loss). This error is then used to update the model’s parameters (weights and biases).
Example: Suppose the model predicted a probability of 0.7 for “cat” and the true label is “dog.” We would calculate the loss using a loss function, like cross-entropy. The loss indicates how far off the prediction was, which we then propagate back through the network.
Illustration:
- True label:
y_true = [0, 1]
(for “dog” ) - Predicted:
y_pred = [0.7, 0.3]
- Loss:
L = - (y_true * log(y_pred)) = - (0 * log(0.7) + 1 * log(0.3) )
- True label:
-
Iterative Optimization: Repeat the forward and backward passes multiple times, gradually refining the model’s parameters until convergence.
Example: During each iteration (epoch), we update the weights using an optimization algorithm like Stochastic Gradient Descent (SGD) or Adam. After many epochs, the model’s predictions should improve, converging towards more accurate outputs.
Illustration:
- Update weights:
W_new = W_old - learning_rate * gradient
- Repeat for a set number of epochs, where in each epoch:
- Forward Pass: Get predictions
- Backward Pass: Calculate loss and gradients
- Update weights
- Update weights:
3. Model Evaluation:
- Use Validation Set: Assess the model’s performance on a separate validation set to avoid overfitting.
- Evaluate Metrics: Choose appropriate metrics based on your task e.g., accuracy, precision, recall, F1-score, mean squared error.
Strategies for Model Training:
- Fine-Tuning: If you have a pre-trained model, you can adapt it to your specific task by training it on your dataset using smaller learning rates. In simple terms, fine-tuning refers to modifying the pre-trained model’s architecture to fit your specific requirements. For example, if you are using a pre-trained model designed for classifying 1,000 objects but your task only involves 10 objects, you can adjust the model accordingly.
- Transfer Learning: Instead of starting from scratch, we can leverage a pre-trained model like ResNet50, which has been trained on the ImageNet dataset containing millions of images. ResNet50 has already learned to extract useful features like edges, textures, and shapes that are likely relevant to our task. By fine-tuning the final layers of ResNet50 on our dataset of dog and cat images, we allow the model to adapt to the specific features that differentiate dogs from cats. This approach typically results in better performance compared to training a model from scratch.
- Regularization: Techniques like L1 or L2 regularization can prevent overfitting by penalizing complex models.
- Early Stopping: Monitor the model’s performance on the validation set and stop training if it starts to deteriorate to avoid overfitting.
Considerations for Limited Data:
- Data Augmentation: Generate more training data from existing samples.
- Transfer Learning: Utilize pre-trained models on similar tasks.
- Simple Models: Consider simpler models that are less prone to overfitting with limited data.
- Regularization: Employ regularization techniques to prevent overfitting.
How to choose a right model or algorithm for your task?
From my personal experience and learning, the best approach to model selection is to first train the model on a small but representative dataset and evaluate its performance. This allows us to determine whether the model is suitable for the specific task before committing to training it on a larger dataset. An additional benefit is that it saves time by helping us quickly decide whether to use a different algorithm, change the model, or revisit necessary preprocessing steps in the data.
In the case of different algorithms like Random Forest, Decision Tree, Logistic Regression, SVM, etc., that can be used for tasks such as heart disease classification or spam detection, I personally prefer using cross-validation to find the best algorithm with higher accuracy.
Example
#Load dataset
data = load_iris()
X, y = data.data, data.target
#Initialize models
model_rf = RandomForestClassifier()
model_svc = SVC()
#Perform cross-validation
scores_rf = cross_val_score(model_rf, X, y, cv=5)
# 5-fold cross-validation
scores_svc = cross_val_score(model_svc, X, y, cv=5)
#Print mean scores
print(“Random Forest Mean Cross-Validation Score:”, scores_rf.mean())
print(“SVM Mean Cross-Validation Score:”, scores_svc.mean())
Is getting a high test accuracy enough?
The most important thing to consider is customer satisfaction by meeting the requirements, rather than just achieving high test accuracy. One common problem we might encounter is that a model may perform well with high accuracy but still be biased.
For example, say we have developed an e-commerce recommendation system that is performing very well but may only target specific users or recommend products from specific retailers.
Lets make it more clear with another scenario
Imagine a self-driving car model that achieves a high accuracy rate in simulated tests. However, when deployed on real roads, it fails to handle unexpected situations like sudden lane changes or adverse weather conditions. This demonstrates the importance of evaluating models beyond test accuracy, considering factors such as generalizability, robustness, and ethical implications to ensure their safe and effective operation in real-world environments.
Error Analysis: Identifying and Addressing Model Flaws
Error analysis is a critical step in machine learning that involves systematically investigating and understanding the mistakes made by a model. By analyzing errors, you can gain insights into the model’s strengths, weaknesses, and potential areas for improvement. Developers often get headache finding the error and fixing it so you can try this out when you tackle error without any headache.
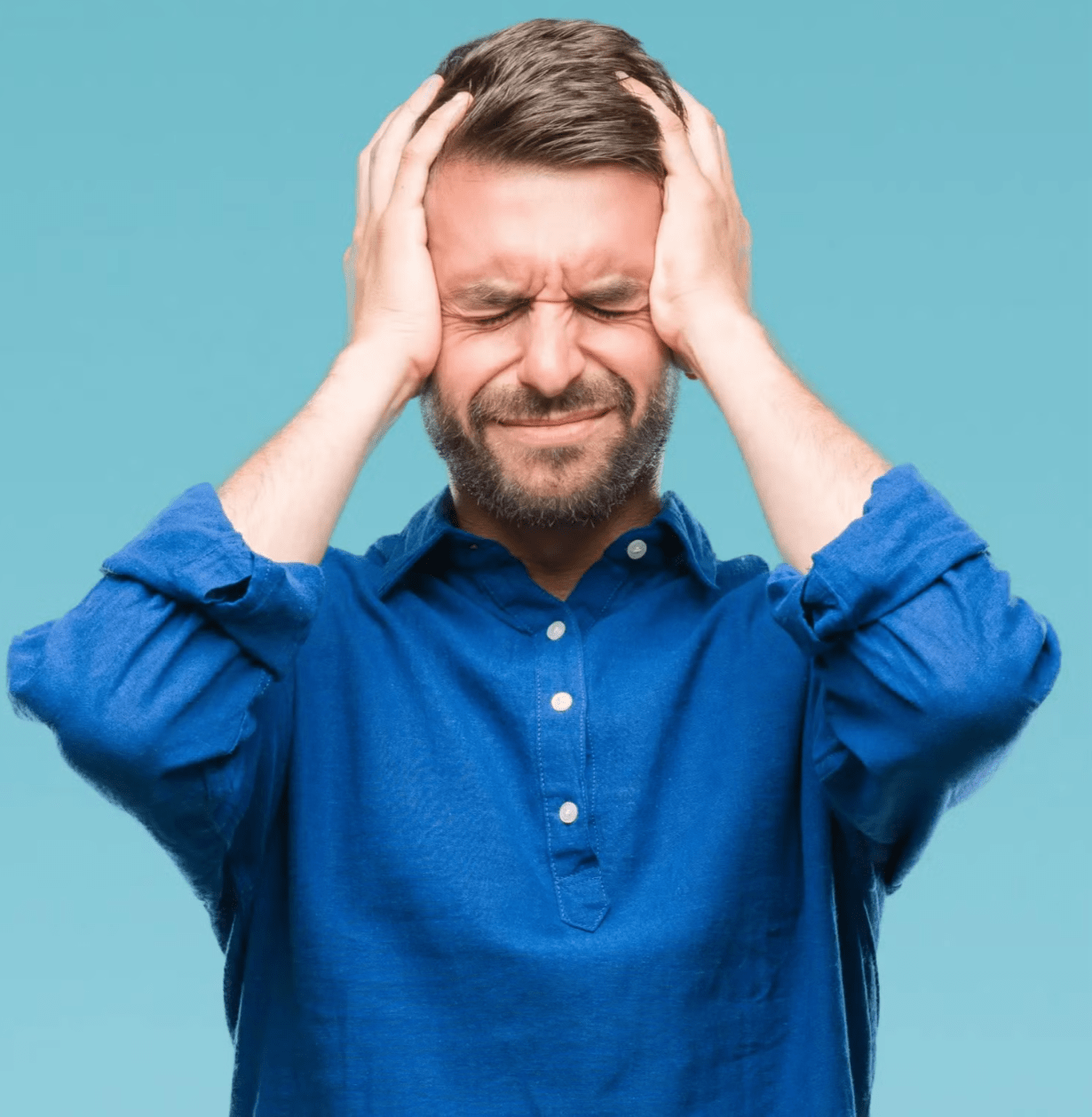
Key Steps in Error Analysis:
1. Identify Errors:
- Compare Predictions and Ground Truth: Analyze the discrepancies between the model’s predictions and the actual correct outcomes.
- Use Error Metrics: Calculate relevant metrics e.g., accuracy, precision, recall, F1-score to quantify the model’s performance.
2. Classify Errors:
- Categorize Errors: Group errors based on common patterns or characteristics. This can help identify specific types of mistakes the model is making.
3. Analyze Error Patterns:
- Explore Root Causes: Investigate the underlying reasons for the errors. Are they due to data quality issues, model complexity, or other factors?
- Identify Bias: Check for biases in the data or model that might be contributing to errors.
4. Visualize Errors:
- Create Visualizations: Use plots, charts, or other visualizations to better understand error patterns and identify trends.
Common Error Types:
- Bias: The model consistently makes errors in a particular direction due to biases in the data or model architecture.
- Variance: The model’s performance varies significantly across different training sets, indicating overfitting.
- Noise: Random errors that are difficult to explain or correct.
Strategies for Improving Model Performance:
- Data Quality: Improve data quality by addressing issues like missing values, outliers, and inconsistencies.
- Feature Engineering: Create or transform features to better capture relevant information.I have explained about this in much detail in part 1(machine-learning-from-ideation-to-production) ,you can check for part 1 for clear understanding.
- Model Selection: Experiment with different model architectures to find one that is better suited to the task.
- Hyperparameter Tuning: Fine-tune the model’s hyperparameters to optimize performance.
- Ensemble Methods: Combine multiple models to reduce variance and improve accuracy.
Conclusion
We have successfully navigated the critical stages of model training ,evaluation, and error analysis. By carefully selecting algorithms, fine-tuning parameters, and assessing performance metrics, we have developed a model capable of making accurate predictions.
Now, it’s time to take the next crucial step: deployment. In the upcoming part, we will explore the strategies and considerations involved in deploying our trained model into a real-world environment. We’ll discuss topics such as infrastructure, scalability, and monitoring to ensure our model operates effectively and delivers value.
Stay tuned as we embark on the final phase of the machine learning project lifecycle!