Next.js Tutorial for React devs
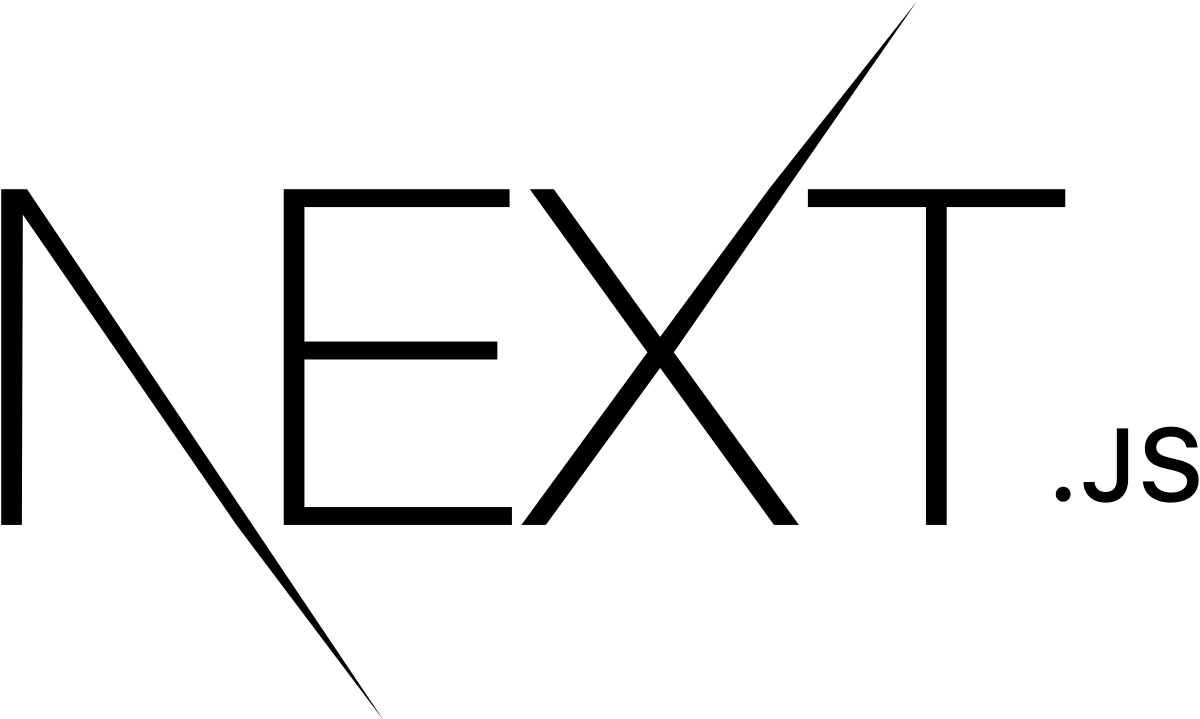
Welcome, React developers! If you’re looking to elevate your React applications to the next level, you’re in the right place. Next.js is a powerful React framework that enables server-side rendering, static site generation, and much more, making it a fantastic choice for building high-performance and SEO-friendly web applications. This tutorial is designed to guide you through the core concepts of Next.js, leveraging your existing React knowledge.
What is Next.js?
Next.js is an open-source React framework that provides infrastructure and simple development experience for server-side rendered(SSR) and statically generated web applications. It includes out-of-the-box features like page-based routing, pre-rendering, and automatic code splitting, helping developers build fast and efficient web applications.
1. Getting started
First, ensure that you have Node.js installed on your system and then create a new Next.js app by running the following command.
npx create-next-app@latest my-next-app
# or
yarn create next-app my-next-app
This command creates a new directory called my-next-app
with a minimal setup. Navigate into your project:
cd my-next-app
You can run your development server by running the following command.
npm run dev
# or
yarn dev
2. Pages & Routing
Next.js uses a file-system-based routing mechanism. That means pages are associated with a route based on their file name in the pages
directory. For example, pages/about.js
is automatically routed to /about
.
Creating a New Page
Create a file called about.js
inside the pages
directory and add the following content:
export default function About() {
return <div>About us</div>;
}
Now, /about
will render this component.
3. Static Generation and Server-side Rendering
Next.js offers two forms of pre-rendering: Static Generation and Server-Side Rendering. By default, Next.js pre-renders pages using Static Generation, which generates the HTML at build time. This method is recommended for pages that can be pre-rendered ahead of a user’s request. However, if you need to render pages on each request, you can use Server-Side Rendering.
Static Generation and Server-side Rendering
Use getStaticProps
to fetch data at build time. For example, to fetch posts and pass them to your page component:
export async function getStaticProps() {
const res = await fetch(‘https://…/posts’);
const posts = await res.json();
return {
props: {
posts,
},
};
}
Server-side Rendering
Use getServerSideProps
to fetch data on each request:
export async function getServerSideProps() {
const res = await fetch(‘https://…/data’);
const data = await res.json();
return {
props: {
data,
},
};
}
4. Project Structure
Let’s dive deeper into how you can structure a Next.js 13 application with layouts, templates, and custom error handling, including examples of code and file hierarchy. This example will showcase how to organize your application using the new app
directory, implement a global layout, nested layouts, and a custom error page.
Static Generation and Server-side Rendering
Use getStaticProps
to fetch data at build time. For example, to fetch posts and pass them to your page component:
export async function getStaticProps() {
const res = await fetch(‘https://…/posts’);
const posts = await res.json();
return {
props: {
posts,
},
};
}
Server-side Rendering
Use getServerSideProps
to fetch data on each request:
export async function getServerSideProps() {
const res = await fetch(‘https://…/data’);
const data = await res.json();
return {
props: {
data,
},
};
}