Performance optimization is a critical aspect of web development, especially as users expect applications to load quickly and respond smoothly to their interactions. In the React ecosystem, optimizing performance not only enhances the user experience but also reduces load times and improves responsiveness. As an application grows in complexity and size, it is essential to focus on performance techniques to maintain its efficiency.
Understanding React’s Rendering Process
To optimize React applications effectively, it is important to understand how React renders components:
- Virtual DOM: React maintains a virtual DOM to track component changes. When a component’s state or props changes, React updates the virtual DOM first
- Reconciliation: React then compares the new virtual DOM with previous ones to identify changes that need to be applied
- Commit Phase: React applies the identified changes to the actual DOM
Now, let’s explore some key techniques for performance optimization.
1. Memoization:
Memoization is a technique for avoiding redundant or expensive calculations by caching results and reusing them when the same inputs occur again. Primary hooks for memoization are:
- React.memo()
- useMemo()
- useCallback()
React.memo()
React.memo() wraps a functional component to prevent unnecessary re-renders by ensuring it only re-renders when its props changes.
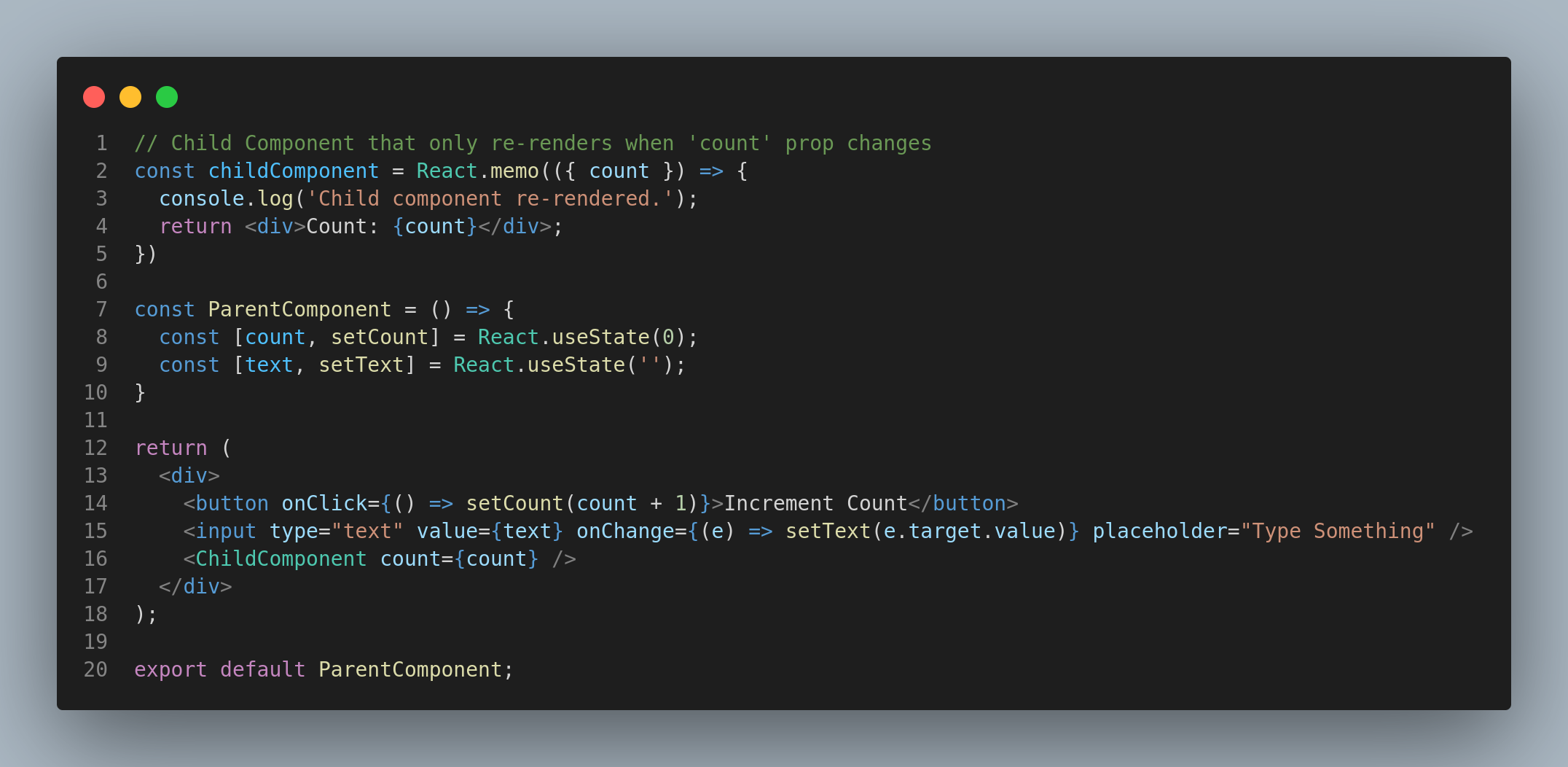
useMemo()
useMemo() memoizes the result of an expensive computation. It only re-computes when its dependencies change, reducing the processing load on subsequent renders.
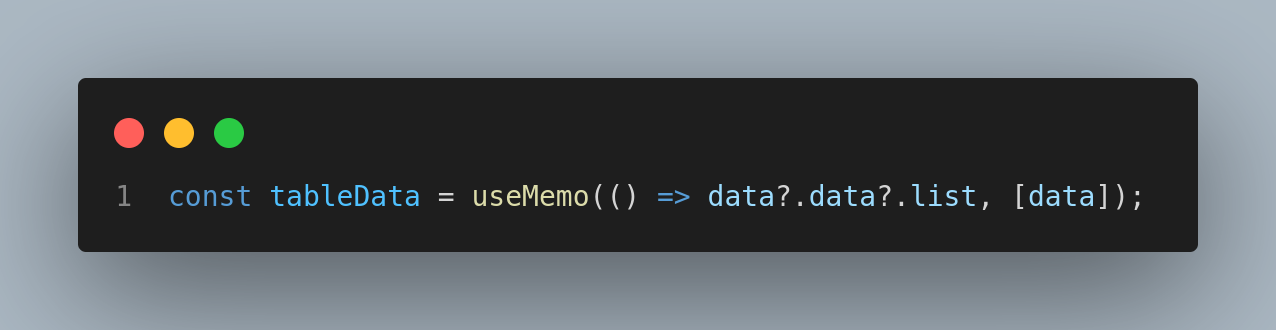
In this example:
Without useMemo(): Expensive calculations are performed on every render, even when dependencies haven’t changed.
With useMemo(): The result is calculated only when dependencies change, and the cached result is returned on subsequent renders, reducing re-renders
useCallback()
useCallback() memoizes a function, ensuring it only changes if its dependencies change. This is particularly useful when passing functions as props to child components to prevent unnecessary re-renders.
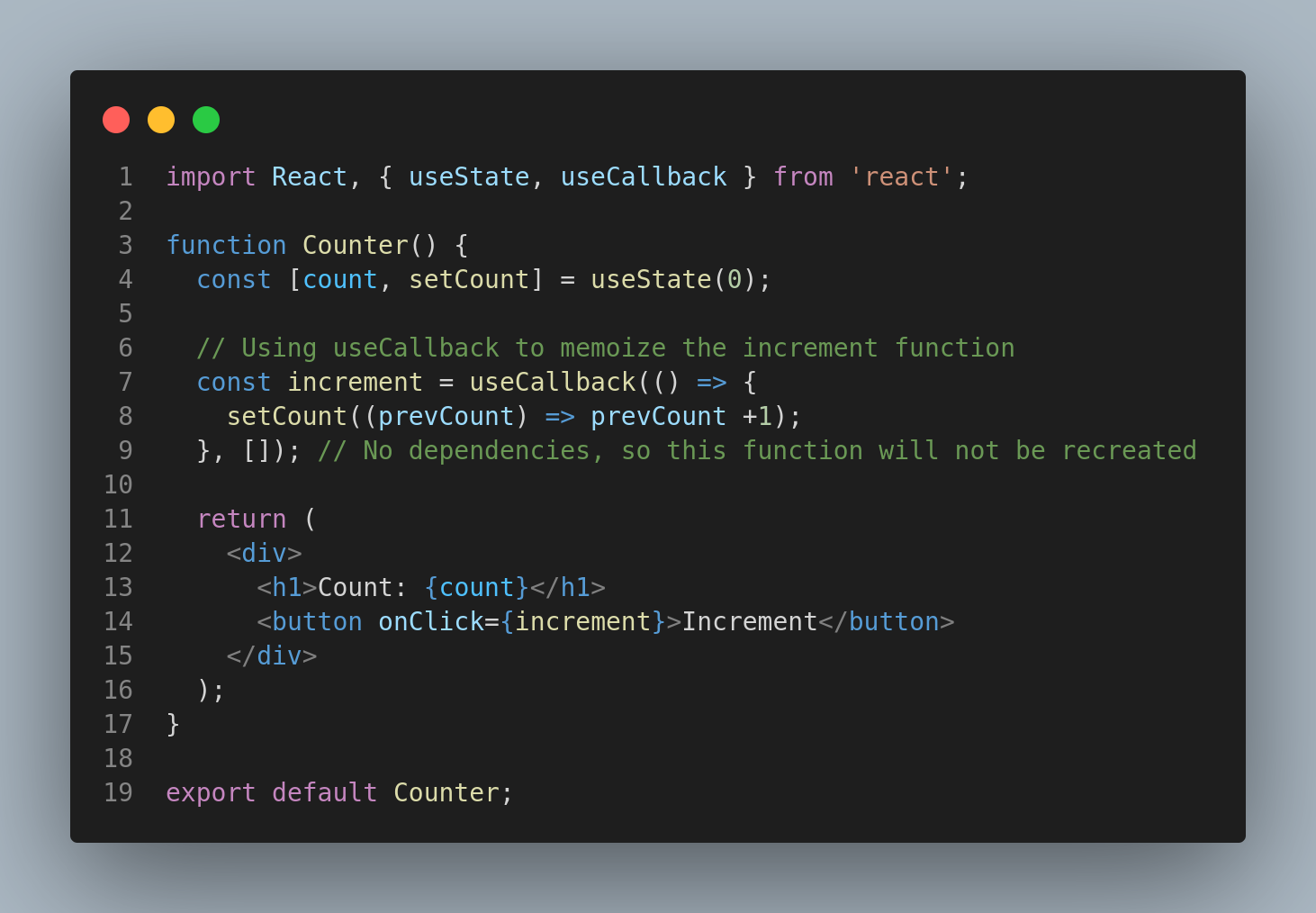
In this example:
Without useCallback(): The Button component would re-render every time the state count changes.
With useCallback(): The onClick function is memoized, ensuring the same function reference is passed to the Button component, preventing unnecessary re-renders.
2. Lazy Loading Images in React:
Lazy loading defers the loading of off-screen images until they enter the viewport, improving the initial load time. React can achieve lazy loading using native browser support or by utilizing a third-party library such as react-lazyload.
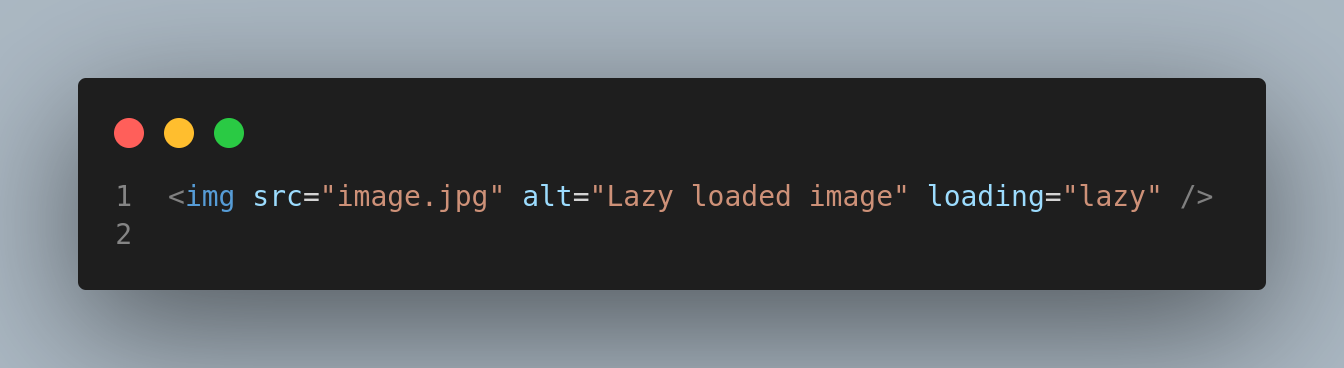
In this example:
With the loading=”lazy” attribute, images will only load when they are about to enter the viewport.
3. Minification of CSS and JavaScript Files
Minification involves removing unnecessary characters (like spaces, comments, and newlines) from CSS and JavaScript files, making them smaller and faster to load. Both Webpack (used in CRA) and Vite automatically minify assets during the production build process.
4. Font Optimization
Using optimized fonts can greatly enhance load times:
- Use modern formats like WOFF2: This format offers better compression than older formats like TTF.
- Preload critical fonts: Ensure that important fonts are loaded quickly using the rel=”preload” attribute.
- Use system fonts: For applications where performance is crucial, using system fonts (Arial, Helvetica, etc.) can reduce the time required to download and render custom fonts.
5. Virtualization for Large Lists
Virtualization (or windowing) renders only the visible part of a list and loads other items dynamically as the user scrolls. Popular libraries include react-window and react-virtualized
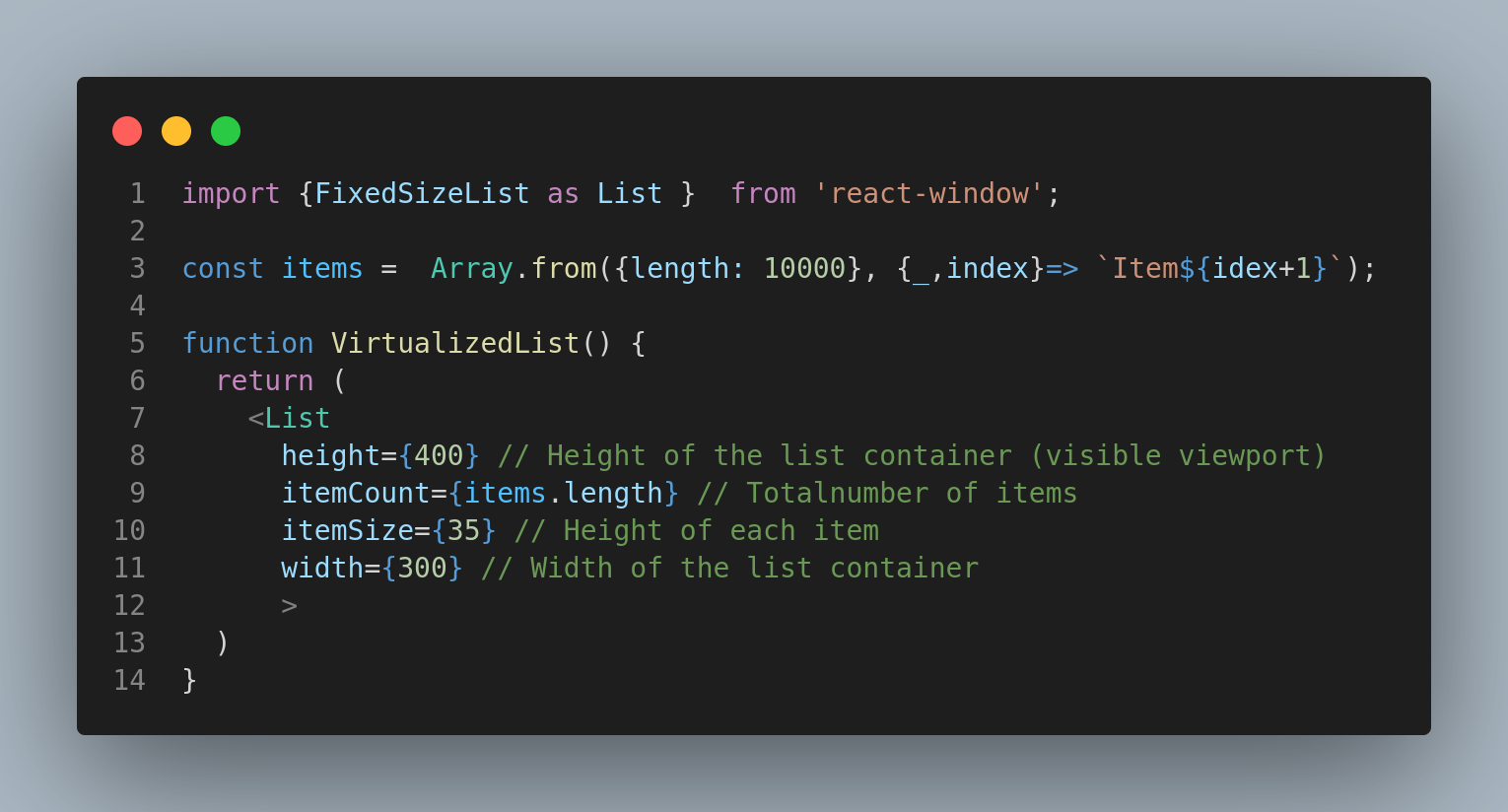
Benefits of Virtualization :
- Reduced Initial Rendering Time: Only a small subset of items are rendered initially, speeding up the page load.
- Improved Scrolling Performance: By reducing the number of rendered DOM elements, virtualization leads to smoother, more responsive scrolling.
- Lower Memory Usage: Virtualized lists only keep a few elements in memory, reducing the overall memory footprint.
- Optimized User Experience: With faster load times and smoother interactions, virtualization helps maintain a consistent and performant user experience even with large datasets.
6. Code Splitting and Lazy Loading
Instead of loading the entire application at once, code splitting loads only the necessary parts, significantly reducing initial load times.
Webpack and Code Splitting
Webpack is the most commonly used module bundler for React apps, and it automatically splits the code into smaller bundles when you use dynamic import() statements or React’s lazy loading.
Dynamic Imports
Webpack creates chunks based on dynamic imports, and these chunks are loaded asynchronously.
Splitting by Route or Feature
You can split your code by routes, meaning different sections of your application are split into different bundles. For example, if you have an admin section or a dashboard that is not immediately needed, you can delay its loading until the user navigates to that page.
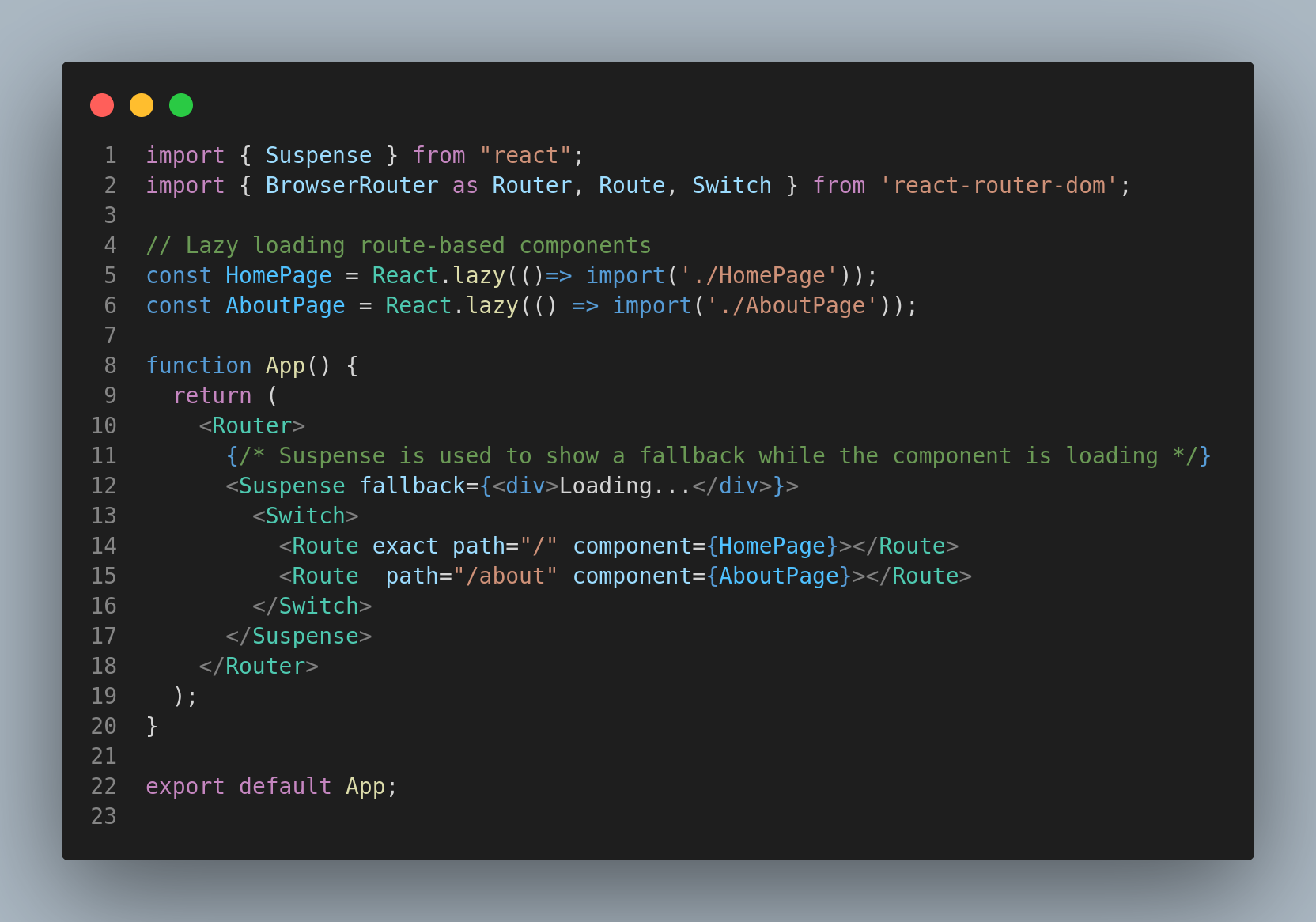
In the example above:
React’s React.lazy() and Suspense React introduced the React.lazy() function to allow components to be loaded lazily, meaning they are only loaded when they are rendered for the first time.
How Webpack Handles Code Splitting
Webpack can automatically split your code into separate chunks. When you use dynamic imports (like React.lazy), Webpack recognizes these imports and creates separate chunks for those components.
Webpack uses two main techniques for splitting code:
- React.lazy(): This function allows you to dynamically import a component only when it’s needed, deferring the loading of the component. This ensures that the initial bundle size is smaller, and additional components are loaded on-demand.
- Suspense: While the component is being loaded asynchronously, React will show a fallback UI (like a loading spinner) until the component has fully loaded. This improves the user experience by indicating that something is happening in the background.
Conclusion
By following these best practices, you can build a React application that’s efficient, responsive, and optimized for performance across a wide range of devices and network conditions. Performance optimization is an iterative process that benefits from constant monitoring and improvement based on user interactions and analytics.